Understanding cybersecurity basics has become an integral part of software development with the rise of the internet and the prevalence of software attacks like ransomware, phishing, or malicious code injections. Without proper cybersecurity measures, any application can be compromised, resulting in user data thefts, financial losses, and even the loss of user trust. This guide aims to help developers avoid many common cybersecurity issues while building secure and resilient applications.
Understanding Cybersecurity Basics
Common Types of Cybersecurity Threats
- Malware: Here, we are referring to viruses, worms, ransomware, and spyware. Such malicious programs often infiltrate systems, steal data, ruin files, and create operational chaos. Understanding how malware works is vital to protecting systems properly.
- Phishing: Phishing is the act of fooling users into giving away personal information, such as usernames, passwords, and credit card details, by pretending to be someone or some trusted entity. It is typically done via email, fake websites, and social engineering.Developers need to educate users on how to spot phishing attempts and employ technical countermeasures to detect and block phishing attacks.
- Man-in-the-Middle (MitM) Attacks: In a MitM attack, an attacker sits between two parties to the communication. As the attacker observes and potentially modifies the communication, both parties are unaware that their communication has been compromised. This can lead to data theft and unauthorised access to parties to the communication. You can help mitigate such attacks by ensuring that the communication is protected by strong encryption protocols, like HTTPS, and by using secure key exchanges.
- Denial-of-Service (DoS) Attacks: A DoS attack aims to render a network service inaccessible by flooding it with requests. It is distributed (i.e., a DDoS attack) if multiple compromised systems are involved. Developers can defend against these attacks by using secure network infrastructure such as firewalls, load balancers, and anti-DDoS services.
- SQL Injection (SQLi): SQL injection involves code injection into a web application’s database layer, exploiting vulnerabilities in the way a database receives and processes input data. This allows attackers to insert queries and extract data they should not be able to access. Defence strategies include employing parameterised queries and stored procedures to sanitise data inputs and prevent injection attacks properly.
Real-World Examples
- Case Studies of Major Cybersecurity Breaches: The Equifax data breach and the WannaCry ransomware attack are two notable examples of the damage that can result from cybersecurity failures. In both cases, sensitive information was exposed, and companies and individuals experienced significant material and reputational loss.
- Lessons Learned from These Breaches: The key takeaways are that patch management must be done in time, every organisation should consider regular security assessments, incident response plans are crucial and must be well-documented, and security can never be too proactive because cyberattacks are constant and unexpected.
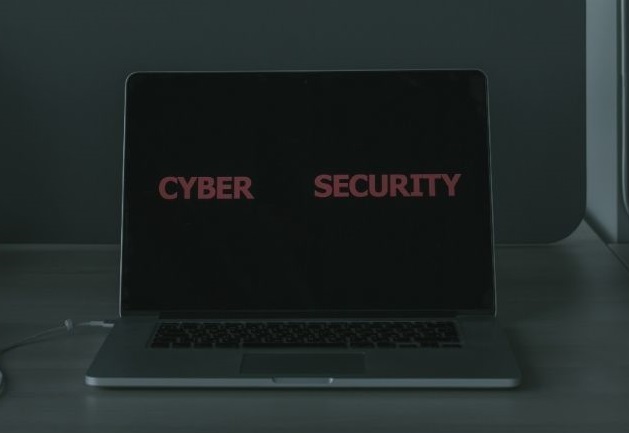
Secure Coding Practices
Input Validation
- The Importance of Validating User Input: Input validation ensures that the data entering your system is clean, accurate, and secure to prevent malicious inputs that could pose security vulnerabilities.
- Input validation techniques: Allowlisting of allowed inputs, built-in validation functions, and regular expressions to validate formats. Input should always be validated on both the client and the server.
Output Encoding
- Setting Data to the Correct Encoding Before Rendering: Before the browser renders output data to the end-user, the output is converted to a format that will not allow cross-site scripting (XSS) attacks.
- Common Encoding Cheatsheets and Libraries: Input or output provided by a developer is typically encoded either by making a call to a library like OWASP ESAPI or through built-in library functions that most frameworks provide (e.g., HTML encoding, URL encoding and CSS encoding): HTML encoding: <div>I’m an XML document!</div> URL encoding: https://www.google.com/search?q=Broken%20URI URL encoding: https://www.google.com/search?q=Broken+URI CSS encoding: body { background-color: #f00; }
Authentication and Authorization
Good Security Habits:
• Use strong, unique passwords.
• Require password complexity (e.g., mixed case, numbers, symbols)
• Use password hashing algorithms such as bcrypt
• Never store plaintext passwords.
Role-Based Access Control (RBAC) – granting permissions based on the roles users play, so users have access only to what they need and no more.
- Multi-Factor Authentication (MFA): What is it, and how does it work? MFA is another level of security after the established password. Implementing MFA the right way involves combining two or more factors: something the user knows (password), something the user has (smartphone), or something the user is (fingerprint).
Secure Storage
- Sensitive Data at Rest: Encrypted data is unreadable to anyone who does not have the decryption key. To safeguard data at rest from unauthorised access, the use of strong encryption algorithms such as AES-256 is necessary for encryption.
- Storing Credentials and API Keys Securely Using Secure Storage Mechanisms: Storing credentials and API keys in source code is a terrible idea. Instead, store them in secure vaults or environment variables. AWS Secrets Manager and HashiCorp Vault are two examples of secure storage systems.
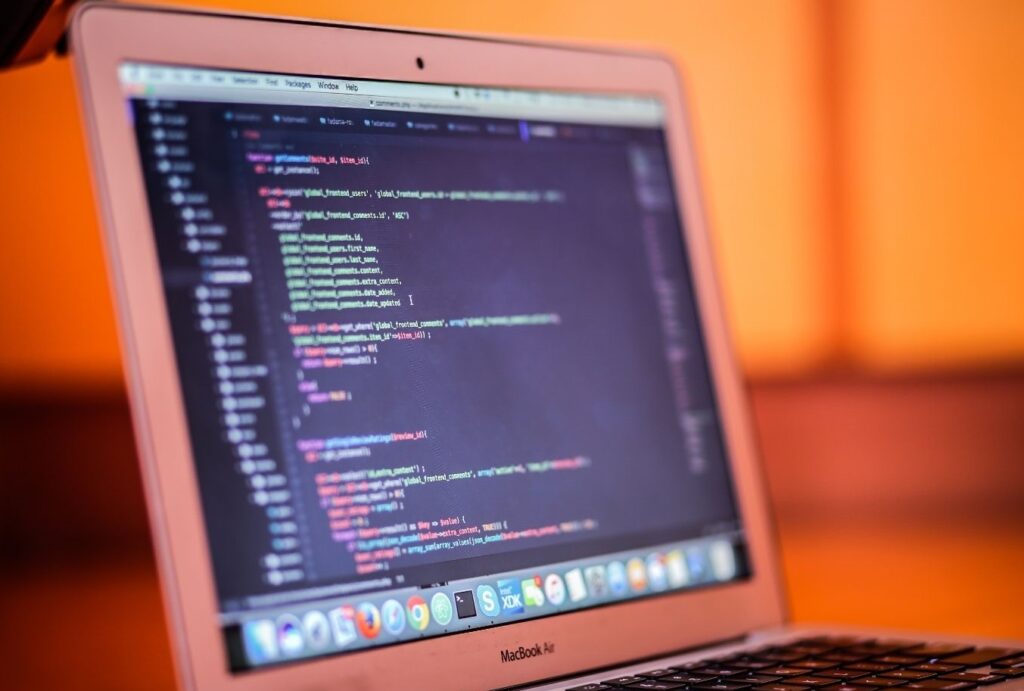
Secure Development Lifecycle (SDLC)
Integrating Security into SDLC
- Phases of SDLC with a Security Focus: Integrate security in every phase of SDLC—from the planning and design of a product to its implementation, testing, deployment, and maintenance. This way, security is embedded in the product at every stage.
- Advantages of Building Security: Building security early and maintaining it throughout reduces the risk of vulnerabilities, and the cost of fixing defects builds a security-first culture and strengthens software quality.
Threat Modeling
- Scoping Threat Model: Identify possible attackers, types of attacks, and targets.
Characterise Assets: Decide what is in scope.
Characterise Threats: Prioritise threats according to impact and likelihood.
Assess Vulnerabilities: Decide what is in scope. - Tool and Techniques for Threat Modelling: DREAD (Damage potential, Reproducibility, Exploitability, Affected users, Discoverability) and similar techniques such as STRIDE (Spoofing, Tampering, Repudiation, Information Disclosure, Denial of Service, Elevation of Privilege). Tools such as Microsoft Threat Modeling Tool and OWASP Threat Dragon.
Code Review and Testing
- Peer Code Reviews: Peer reviews are an excellent way of catching security flaws early in the development process, which helps prevent vulnerabilities from reaching the finished product. Peer reviews can involve inspecting the code for security issues or making sure that the code follows all of the best security practices.
- Automated Security Testing Tools: These include static application security testing (SAST) to detect vulnerabilities in the code itself and dynamic application security testing (DAST) to detect vulnerabilities within running applications.
- Security-focused testing (e.g., penetration testing): This kind of testing simulates real-world attacks that try to penetrate your application and systems. For example, by regularly conducting security tests against your application, you can make sure it remains secure as threats change over time.
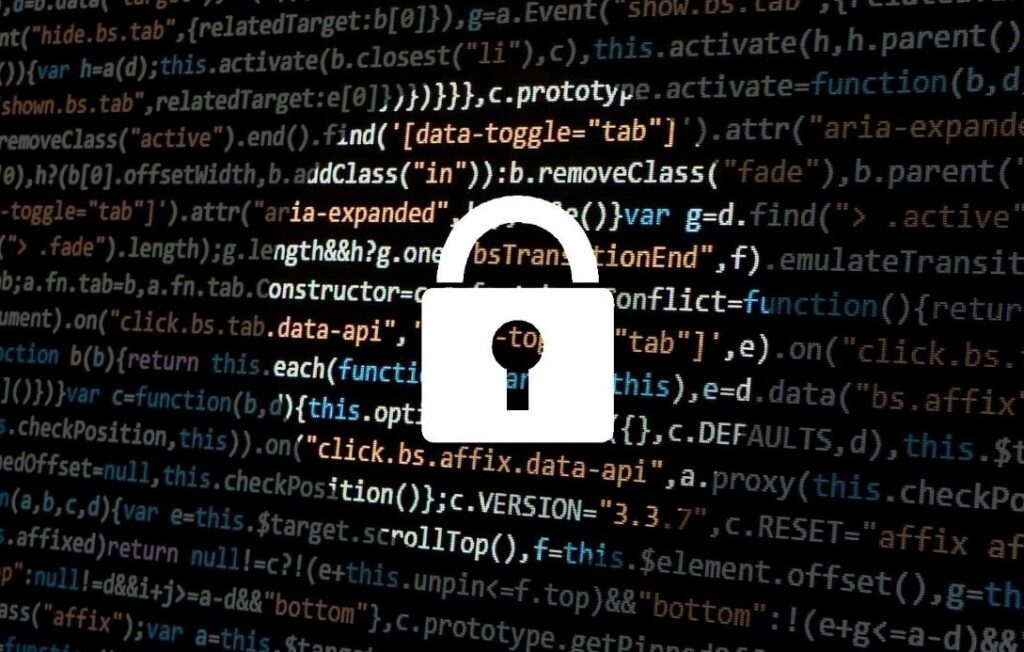
Using Security Tools and Libraries
Static Application Security Testing (SAST)
- SAST Tools: SAST tools analyse source code, bytecode, or binary code to identify security vulnerabilities. They identify potential issues at the earliest stage of development, allowing developers to fix problems before they’re deployed.
- What SAST Tools do: They inspect the internal code structures (without executing them) and identify coding errors, bugs, and security vulnerabilities like SQL injection, XSS, and insecure configurations. Examples of SAST are Checkmarx, Fortify, and SonarQube.
Dynamic Application Security Testing (DAST)
- What are DAST Tools: DAST tools test applications while they run to check for security vulnerabilities. DAST tools are similar to SAST tools, but instead of examining the code, they interact with the application to uncover problems that might not be apparent in static analysis.
- Advantages of DAST: DAST (Dynamic Application Security Testing) tools can identify a runtime vulnerability even if it is not visible during development or staging. For instance, DAST can detect any authentication issues, server misconfigurations, or vulnerabilities that manifest only during runtime. Some examples of DAST tools are OWASP ZAP and Burp Suite.
Dependency Management
- Managing Third-Party Libraries And Dependencies: Building modern applications often means incorporating third-party libraries and frameworks. While using these dependencies can greatly speed up development, they can also introduce vulnerabilities.
- Tools for Vulnerability Scanning in Dependencies (e.g., OWASP Dependency-Check): Tools such as OWASP Dependency-Check and Snyk scan for known vulnerabilities of third-party libraries and help developers maintain and update dependencies to reduce risks.
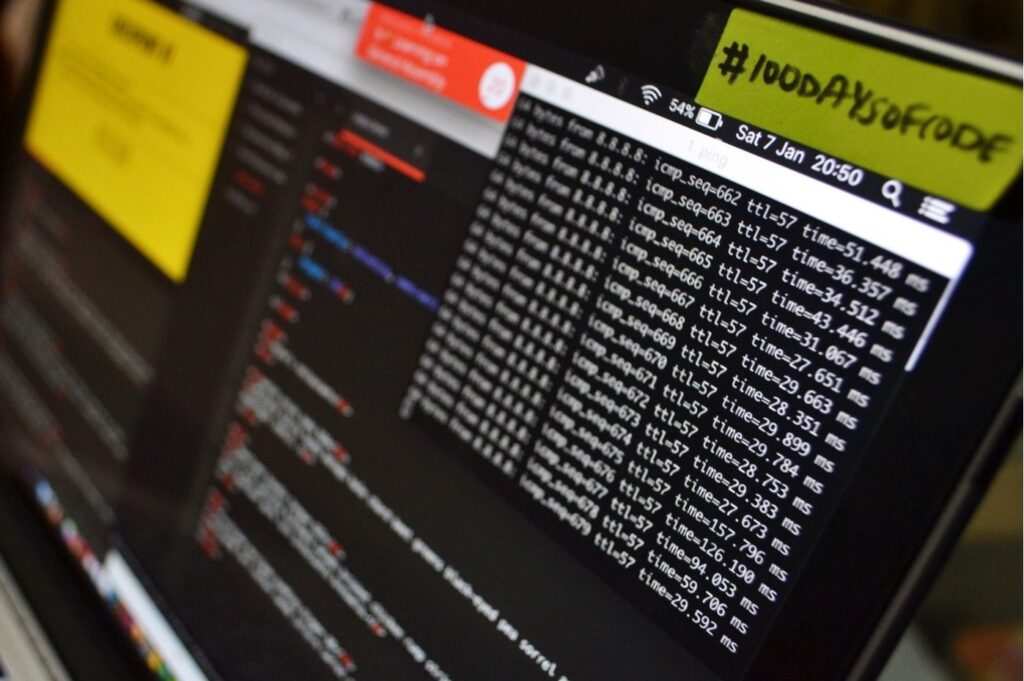
Network Security Fundamentals
Understanding Network Security Basics
- Why Secure Network Communications is Important: Network communications security ensures that data during transmission remains secure from eavesdropping and tampering. This way, data during transit remains confidential and unchanged.
- Standard Network Security Protocols: (e.g. TLS/SSL HTTPS) Standard Network Security Protocols: (e.g., TLS/SSL, HTTPS) TLS/SSL protocols provide encryption over a computer network and protect against eavesdropping and man-in-the-middle attacks. HTTPS provides secure communication between web browsers and servers.
Firewalls and Intrusion Detection Systems (IDS)
- How Firewalls Protect Applications: Firewalls control incoming and outgoing network traffic based on pre-defined security rules. Firewalls are placed between a trusted network and an untrusted network. They discard malicious traffic to protect the trusted network.
- Overview of IDS and How They Function: Intrusion Detection Systems (IDS) observe network traffic for suspicious activities and potential threats. They can be network-based or host-based and alert an administrator in case of a security event.
Secure APIs and Web Services
- Good API practices: To secure APIs, it’s important to use strong authentication and authorisation, use HTTPS, and validate input to prevent injection attacks.
- Rate Limiting and API Authentication: These can help prevent abuse (e.g., limiting the number of requests that a client can make in a given period) and/or ensure that only authorised users can use the API (e.g., using tokens or API keys).
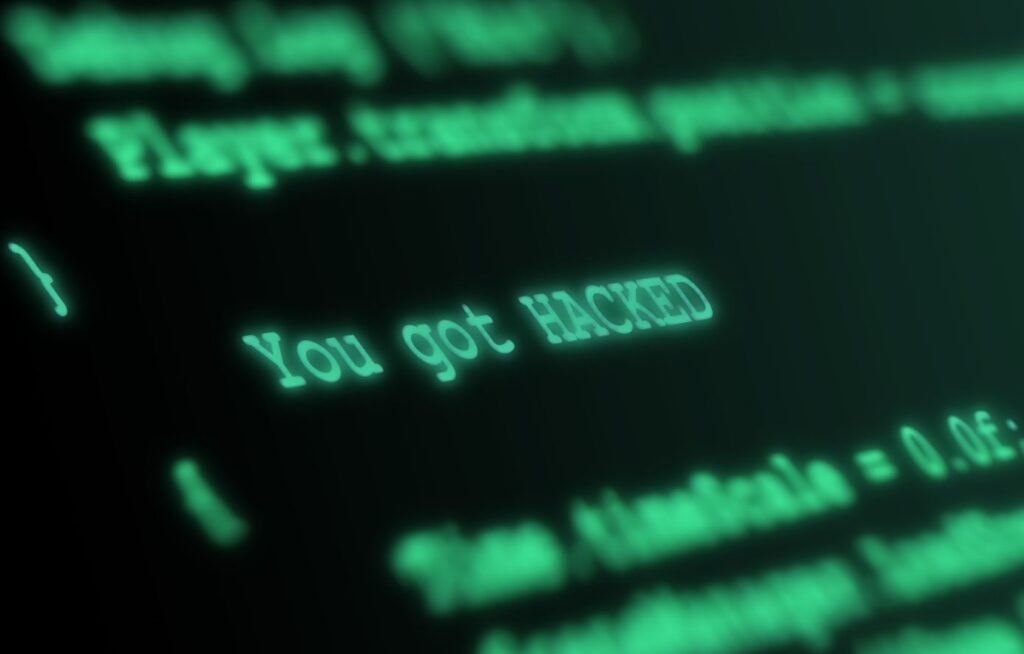
Incident Response and Monitoring
Preparing for Security Incidents
- Building an Incident Response Plan: An incident response plan outlines a consistent, repeatable process for responding to a security breach, including who is responsible for what tasks, how the company will communicate with stakeholders, what needs to be done to contain and eliminate the problem, and how to recover.
- Roles and responsibilities: When someone knows their role, they know what to do. This helps you respond to a security incident quickly.
- Benefits of monitoring applications and systems: It can detect a security incident immediately and take action against potential threats. It can also help identify suspicious activities that indicate that an attack is already underway.
- Security Monitoring and Logging Tools: Examples of these are Splunk, ELK Stack (Elasticsearch, Logstash, Kibana), and Nagios, which collect and log events from multiple sources and generate reports to help spot anomalies.
Post-Incident Analysis
- Post-Mortem: Do a thorough post-mortem analysis after the incident to understand what happened, how it was handled, and how it can be prevented in the future.
- Learn the Lessons Learned to Prevent Another Incident: After an incident, the lessons learned are used to enhance security measures, refine the response and recovery plan, and instruct the team so they know what to expect the next time.
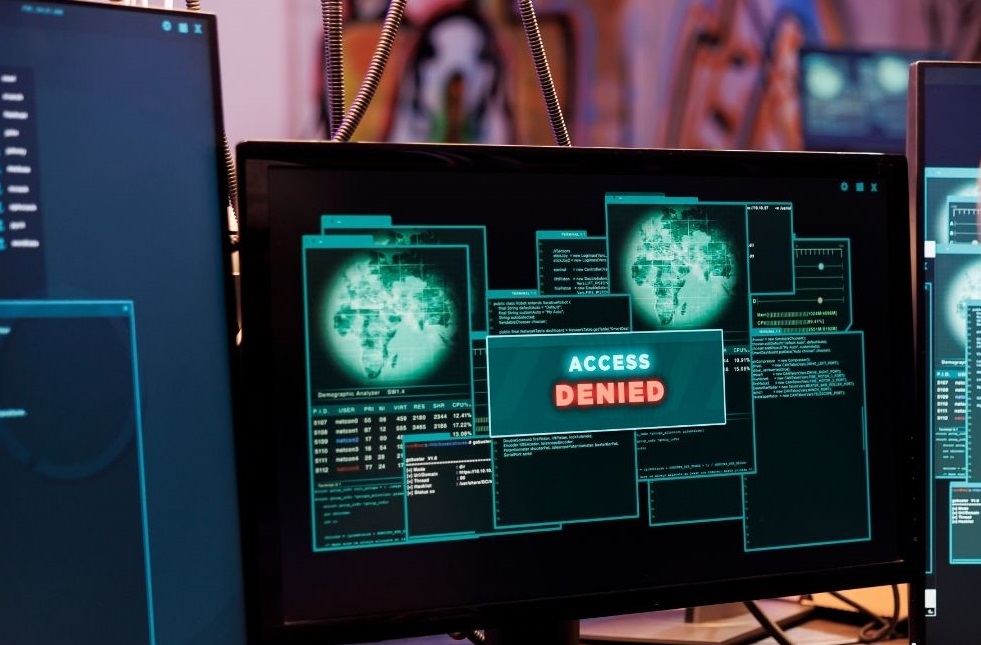
Staying Updated with Cybersecurity Trends
Continuous Learning and Development
- Importance of Continuous Learning in Cyber Security: The cyber security sector is full of innovations; new attacks and weaknesses are discovered every day. Learning practices are a way for developers to keep up to date with the latest developments.
- Resources: To Stay Updated (e.g., Cybersecurity blogs, Courses online, Certifications): cybersecurity blogs (Krebs on Security, Dark Reading), courses online (Coursera, Udemy), certifications (CISSP, CEH)
Community Involvement
- Participating in Cybersecurity Communities and Forums: Cybersecurity is a community effort. Participating in communities and forums such as Stack Overflow, Reddit and cybersecurity-specific forums is a great way to share knowledge, get help and find out about the latest trends and best practices.
- Going to Conferences and Events: Conferences and events such as Black Hat, DEF CON, and the RSA Conference are great places to learn from experts, network with peers, and test new tools and technology.
Conclusion
Developers must use cybersecurity to protect the application and the users from emerging threats. The best practices in cybersecurity must be adopted and maintained to protect data and systems. People have to keep learning the trending stuff and the technology to be advanced in the cybersecurity world.