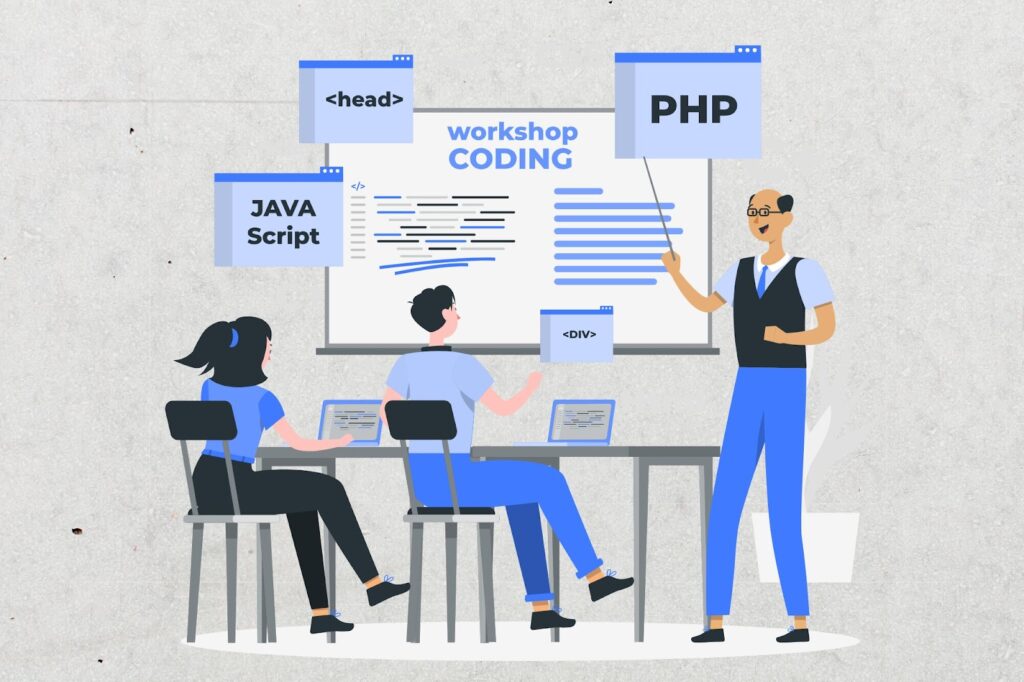
Modern software development almost always relies on efficient coding, which is essential for meeting project deadlines, maintaining code quality, and maximising the performance of the software. When developers do not code efficiently, they tend to experience longer debugging sessions, more complex scaling, and unnecessary delays that can burden the project heavily and compromise both the development and release of the end product. In this blog, we examine every essential tool and technique that a developer should master, from basic tools to advanced methods, which all work towards making the process of coding more efficient and productive.
Integrated Development Environments (IDEs)
Overview of Popular IDEs:
Among all the decisions a developer makes, choosing the right Integrated Development Environment (IDE) is one of the most important because it can have a profound impact on coding efficiency. An IDE actually refers to a sophisticated code-writing tool that provides a complete environment for the developer to write, test, and debug code. Some of the most popular IDEs include Visual Studio Code, IntelliJ IDEA, and PyCharm. Each IDE provides different features that are specifically tailored to the needs of different programming languages and coding methods.
Visual Studio Code, for instance, is very popular due to its flexibility, plugin architecture that allows developers to tailor their environment to many different coding languages and frameworks, and a large library of extensions. IntelliJ IDEA is popular among Java developers for its smart coding help, deep code awareness, and refactoring. PyCharm is popular among Python developers because of its features, such as integrated debugging, testing, and integrated support for Django and other web frameworks.
These IDEs facilitate the coding process by providing features such as syntax highlighting, which makes the code more readable – thus helping to spot errors quickly – and auto-completion, which removes the need for typing repetitive code and reduces the likelihood of making mistakes. The IDEs also provide built-in debugging tools that allow developers to correct problems while they work on them, further increasing the efficiency of development. By providing a full set of tools within one interface, developers can stay concentrated and be more productive, hence making coding more efficient.
Maximising IDE Efficiency:
To really take advantage of the IDE, developers need to go beyond the surface-level features and look deeper into what the IDE is capable of – including ways to customise the settings involved. This way, it can be tailored to your coding style and workflow. For example, you can tweak font size, themes, and key bindings for a more comfortable coding environment, preventing eye fatigue, or create file templates that can save time on common code structures.
Plugins are another important way to make IDEs more productive. Git integration plugins (such as GitLens for Visual Studio Code) let you manage version control from your IDE, making it easier to see what’s changed and who changed it, as well as to collaborate with team members. Linters (such as ESLint for JavaScript or Flake8 for Python) help catch errors and enforce coding standards early in the process before a lot of debugging is done. Code snippet managers (such as Snippets for IntelliJ IDEA) let us type in a common phrase (like if or for) and have the beginning of a snippet (such as ‘if (condition) {‘ or ‘for (var i = 0; i < n; i++) {‘) inserted into our code for us as if we’d just typed it all in.
A quick way to become more efficient is to learn keyboard shortcuts. The majority of IDEs have a large number of shortcuts for common actions such as opening files, moving around the codebase and running tests. Learning these shortcuts is a time-saver because it cuts down on the time spent moving the mouse and navigating through menus. By utilising these features, developers can make their coding process more efficient by reducing the chance of errors and quickly producing higher-quality code.
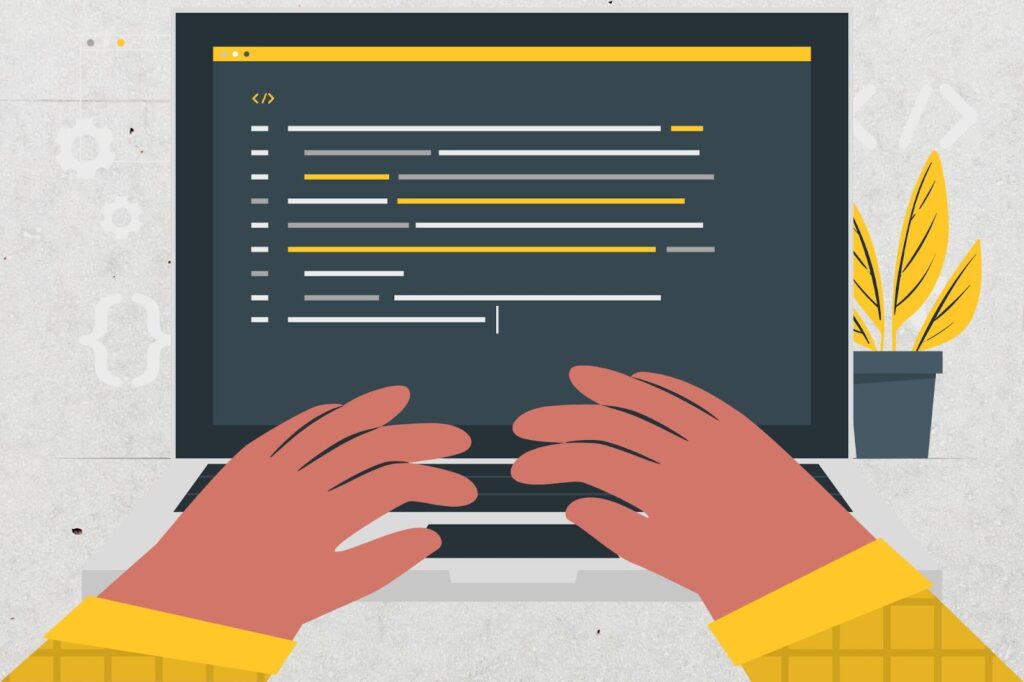
Version Control Systems
Importance of Version Control:
Version control systems (VCS), such as Git, are essential to coding efficiency. They play a crucial role in software development because the codebase of a project is constantly changing. With millions of lines of code, it’s quite easy to lose track of who changed what and when. A VCS helps to track those changes. It keeps a record of all the versions of a project, so developers can easily go back if they need to and make comparisons between versions. This is especially useful in larger projects where many developers are working on the same code at the same time. VCS also helps avoid conflicts when writing code. For example, when one developer is working on one version, and someone else is working on another version simultaneously, it could cause problems.
Having a VCS also means you can revert to previous versions of the code. If a new feature or update causes bugs or performance issues, you can roll back to the last stable version, so no one has to wait around while software bugs are fixed, and no one has to spend extra time diagnosing and solving those problems. Your VCS also helps to document what’s going on — each change includes a commit message, providing a detailed history of the project. This not only makes it easier to debug but also explains the ‘why’ of the code at any given time, which can make the codebase easier to work with in the long term.
Best Practices with Git:
Git is a tool, and, like all tools, it can be used badly. One of the best practices for using Git efficiently, that is, leveraging its strengths while mitigating its weaknesses, is to write good commit messages. Each commit should represent a coherent unit of work, whether that’s adding a new feature or fixing a particular bug; the message should briefly describe what that commit does and what changes it introduces. This makes it easy to track what’s changed and how, especially with a team.
Branching well is another. Building separate branches for features or tasks lets developers work on multiple aspects of a project and merge them without disrupting the main codebase – a feature that’s essential for working on multiple features at once. When a feature is complete, it can be merged back into the main branch. This ensures that only stable code goes into the project. Merge conflicts are an unfortunate but unavoidable reality of using Git, and learning to manage them well is essential. Git comes with several tools for handling merge conflicts, and there are third-party tools like Beyond Compare that can help, too.
Becoming proficient with the most common Git commands will also boost efficiency. Most IDEs include a graphical interface for Git, but command-line Git can often be easier and faster to use for fine-grained control of version-control operations. Commands such as Git rebase, git stash, and Git cherry-pick can be particularly useful for maintaining a streamlined and uncluttered workflow in complicated scenarios. If developers are able to follow these best practices, Git will become a powerful tool that leads to more efficient and well-organised coding.
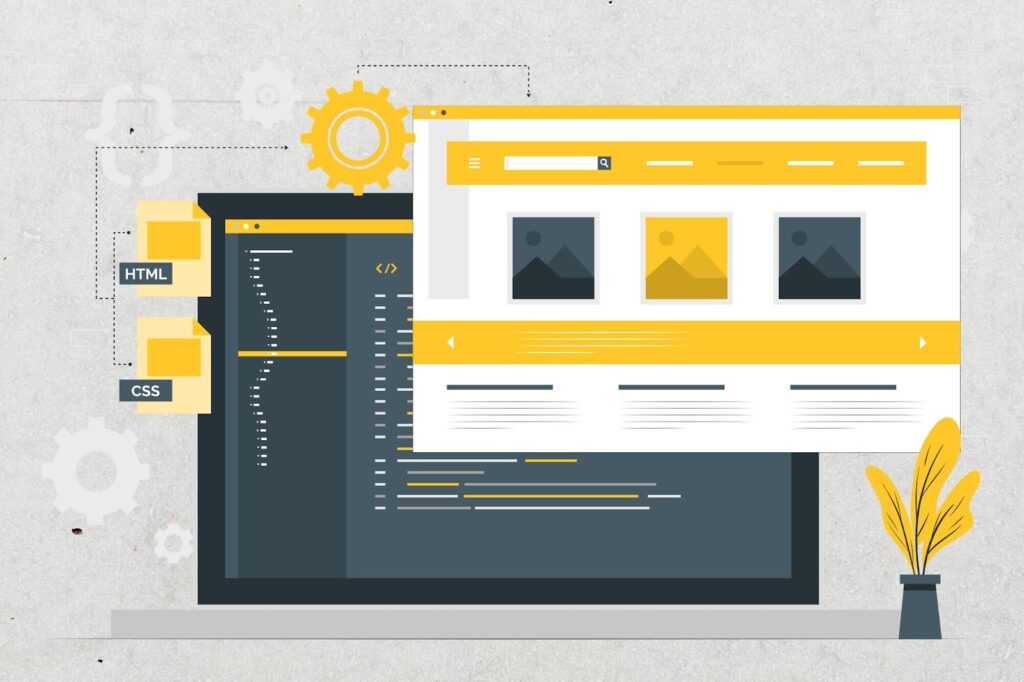
Code Refactoring Tools
The Role of Refactoring in Efficient Coding:
Code refactoring is the process of restructuring an existing body of code without changing its external behaviour. The key goal of refactoring is to make the code better; that is, more readable, maintainable and performant. While the code is being written, we need to be more focused and develop more efficient ways to perform tasks. But, especially as the project grows and its codebase expands, it can become easy to get sidetracked and accumulate redundant or obsolete code. Refactoring is necessary to avoid a tangled mess of spaghetti code that no one can read or modify. Regular refactoring is, therefore, essential to ensure that the code remains clean and scalable.
Third, refactoring is a way to mitigate technical debt. Under tight deadlines, developers might implement a quick fix or workaround (e.g., a ‘hack’), which works but might not be the most efficient solution. Over time, these can accumulate, causing a bloated codebase that’s hard to maintain. Periodic refactorings can address these issues, improving the quality of the software and making it easier to add features or fix bugs in the future. Finally, refactoring can improve the software’s performance, for example, by optimising algorithms or data structures to achieve faster execution times and lower resource consumption.
Popular Refactoring Tools:
There are tools to help developers refactor, making this process faster and error-free. One of the most popular tools among .NET developers is ReSharper. It provides:
- A huge number of refactoring opportunities.
- Starting from renaming a variable.
- Ending with the extraction of a method.
It also extends Visual Studio IDE with additional features by analysing your code in real time and suggesting corrections that improve code structure. Additionally, it provides a navigation system for developers to search for and refactor code in large projects easily.
The second tool I highly recommend is SonarQube, especially when you are working with a large team. SonarQube will run all the time on your codebase, showing you potential problems, including duplicated code, code smells, security issues and so on. SonarQube generates reports and suggests different ways you can refactor your codebase to improve its quality. It is also possible to connect SonarQube with a continuous integration (CI) pipeline so that it will check the quality of your codebase at every stage in the development process.
Automated refactoring support in IDEs such as IntelliJ IDEA or PyCharm also plays a critical role. They enable more complex refactorings that can be done with just a few clicks. For instance, automated tools can be used to rename variables or methods, move classes or extract code into new methods or classes. They automatically update all references, making the process much safer. This helps to keep the code in good shape and maintains high efficiency throughout the development lifecycle.
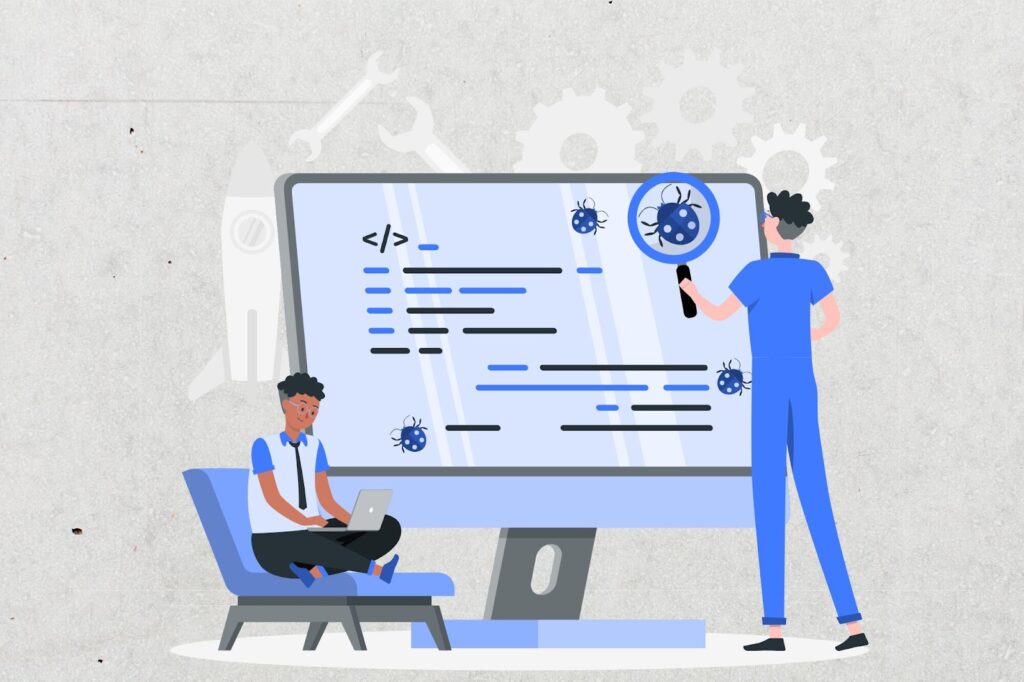
Debugging Techniques and Tools
Efficient Debugging Practices:
Debugging is one of the most important things to do since the ability to recover from bugs prevents what would otherwise be a death march of delays and frustration. A well-tuned debugging practice can help to find and fix the problem quickly and get the development back on track. One of the oldest and simplest debugging practices is logging – which is the act of inserting log statements into the codebase. Log statements can tell you when some part of the program has been executed, what values variables had at that point, and so on. Logging statements are especially useful in nontrivial applications where errors might not manifest themselves directly and can be useful for event monitoring during post-production.
A third important practice is breakpoints: developers can set breakpoints that stop the execution of their code and inspect the state of the application. This is very powerful for the debugging process, as it allows us to see what happens in real time. Often, it is easier to catch a bug by observing it as it happens rather than just by reading the code from top to bottom. If a bug occurs, the developer will see it right when it happens, so it is easier to catch the error.
Another good practice is that of writing unit tests, which can drastically improve debugging efficiency. A unit test is a type of test that tests small components of the software in isolation in order to verify that they are working correctly as standalone units. For instance, if we have a system where a certain component is responsible for connecting to a database, then we should write a unit test that tests how that specific component interacts with a mock database without anything else being involved. By writing good and complete unit tests, we can catch many bugs as we write code before they even end up in the debugging stage. This will significantly reduce the number of bugs that make it to the main codebase.
Top Debugging Tools:
Alongside these efficient debugging techniques, we can employ a number of powerful tools to help us identify and pinpoint problems so that we can fix them sooner. The most widely used debugging tool is GDB (GNU Debugger) – a must-have for anyone developing in C and C++. GDB can halt the execution of a program at an arbitrary point and inspect the state of the program; it can also change variables’ values while the program is running. It supports advanced debugging scenarios, such as applications with multiple threads of execution, and is the go-to tool for debugging at the lowest levels.
Chrome DevTools is a must-have for any front-end developer. A suite of tools integrated directly into the Chrome browser comes with a JavaScript debugger, a network monitor, tools for inspecting and modifying the DOM and CSS, and much more. With Chrome DevTools, you can step through JavaScript code, set breakpoints, and inspect network requests to figure out what’s going on in real time. It’s accessible and has incredibly powerful features, making it the tool of choice for debugging the front end.
They also provide built-in debuggers that are helpful for debugging, like Visual Studio Code, IntelliJ IDEA or PyCharm. Instead of having to switch to different windows, debugging can be done right in the same window where the developer writes the code. They can set breakpoints, look at the values of variables and step through the code all in the same place where they write their code. Such tools significantly reduce the time required to debug code – and the time spent before you can actually write the code.
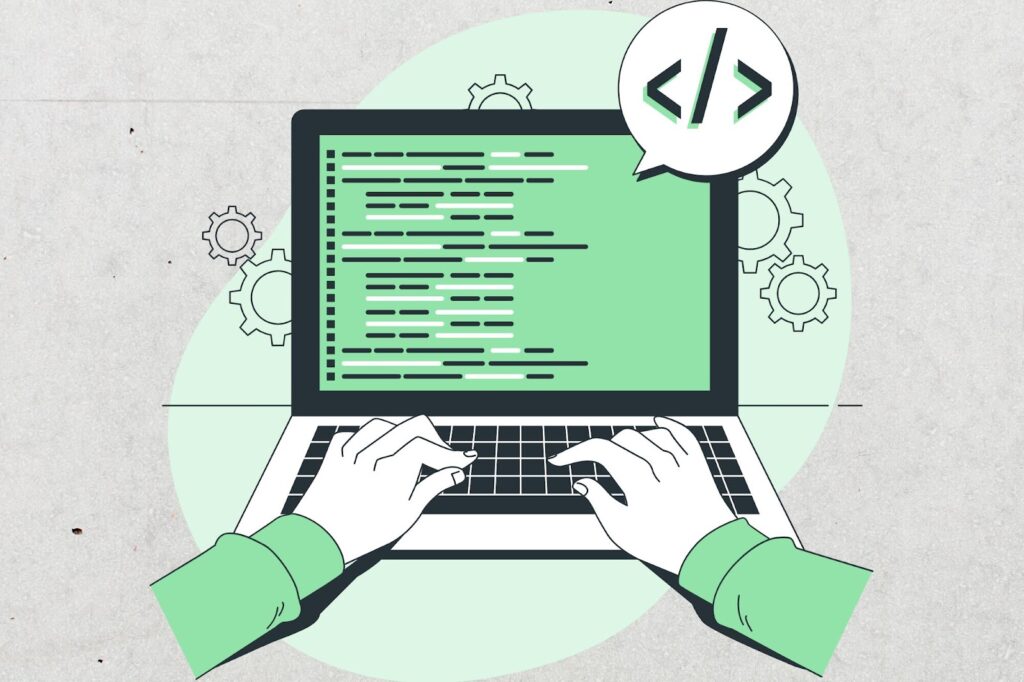
Code Review Practices
The Value of Code Reviews:
Code reviews are a foundational part of effective coding. This essential gate slows down the process of getting things done. Still, it ultimately saves time by catching bugs early, enforcing code quality and serving as an opportunity for knowledge transfer between members of a development team. A thorough code review can uncover some of the problems that automated testing might bypass, such as logical errors, inconsistencies in code style or adherence to coding standards. By catching these problems before they find their way into the main codebase, code reviews help prevent the accumulation of technical debt, saving time and effort that would otherwise be spent on extensive refactoring after the fact.
On top of fostering better code, code reviews help developers learn from each other. For instance, when you review a peer’s code, you get to see how another developer solves the same problem you just worked on or a problem completely remote from the one you’re working on, which helps you pick up new techniques or become familiar with another part of the codebase. This also creates a cooperative environment that encourages a process of self-improvement among the team. In fact, by fostering the exchange of ideas among developers, code reviews help make the entire team more competent, which in turn enables more effective and efficient development. Finally, code reviews also act as a way of enforcing the application of coding standards and best practices because everyone has to follow the same rules when submitting new code for peer review.
Tools for Code Reviews:
Many tools make code reviews easier and more efficient, each with its features and niceties designed to fit particular workflows and team structures. GitHub’s pull requests are one of the most widely used code review tools (especially in open-source projects), where developers can propose changes to a codebase that others can then review on the team before the change is merged into the main branch. This can make it easier to discuss and collaborate on the code while also helping to ensure the code is of high quality and that no changes were made without being discussed or reviewed.
GitLab’s merge requests also offer a unified experience for reviewing and merging changes and include options such as inline comments, suggested code changes and automatic execution of tests as part of the review process. The Review App helps eliminate problems early on in the process. Other popular tools for code review include Review Board (a fork of the original Subversion tool) and Crucible. These tools have many options for managing and tracking reviews, including detailed reports, threaded discussions and integrations with many version control systems. These tools are well suited to larger teams or projects that have more complex review needs. The Review App helps eliminate problems early on in the process.
With these tools, developers can perform more effective and efficient code reviews and merge only the best code that enters the project. Not only will this improve the quality of the software, but it will also help developers maintain a consistent flow in their development, leading to higher productivity and better project outcomes.
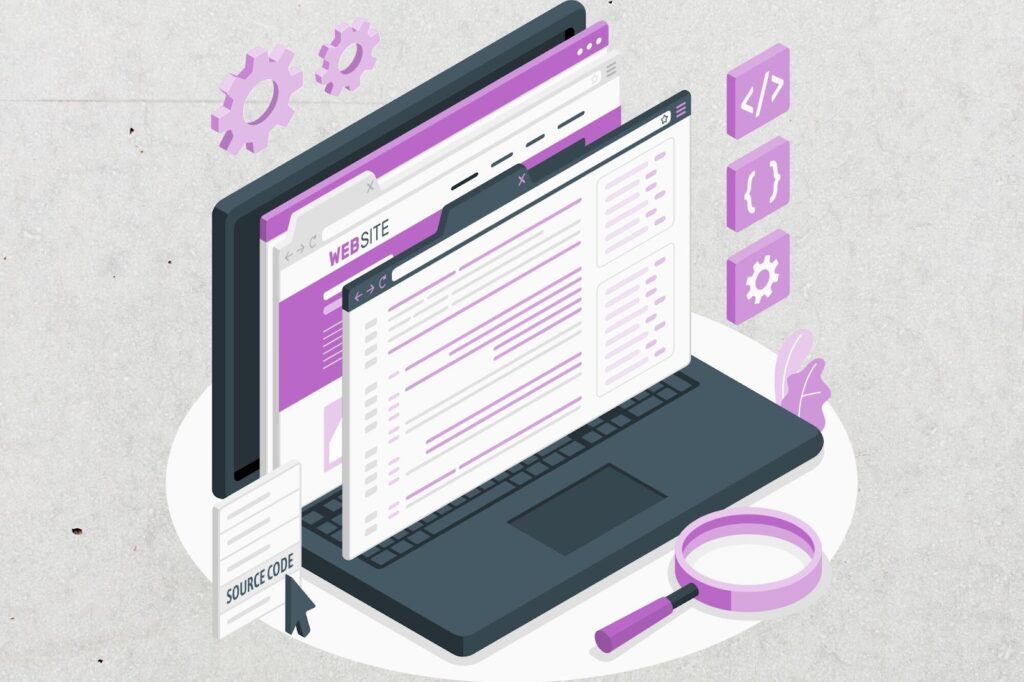
Automated Testing and Continuous Integration
Automated Testing for Efficiency:
Automated testing, in particular, is an integral part of efficient software development: it serves as an early warning system for detecting bugs. It helps maintain the stability of code over time. The issue with manual testing is that it is painstaking and has a high degree of variability. While one tester might be thorough and diligent, another might need to catch up on obvious mistakes. Running automated tests for a given piece of code is fast and consistent, and the results are clear and immediate: one can easily tell when the code is behaving as it should and when it is not. By incorporating automated tests into the development process, developers can ensure that their code is of a certain standard to begin with and minimise the potential for defects to appear later.
Unit tests check the output of a single component in isolation to make sure that it behaves as expected. Integration tests make sure that two or more components work together correctly. End-to-end tests simulate real usage: for example, a shopping cart application will be tested with a set of inputs that would be expected from a normal user. The suite of automated tests catches a wide range of issues before the software gets to the user, which allows developers to deliver software faster and with fewer errors.
Continuous Integration Tools:
Continuous integration (CI) is a software development practice whereby code changes are merged into a shared repository multiple times a day (or even more frequently). Once integrated, the code is tested and built automatically by automated build tools such as Jenkins, Travis CI and CircleCI. Each code change is tested automatically and – assuming it passes the automated tests – becomes part of the main codebase immediately. This way, bugs are caught early in the development cycle before they find their way into the main codebase. It’s a much safer development process than one where code changes are tested in batches long after they’ve accumulated in the main codebase.
Jenkins is one of the most widely used CI tools and undoubtedly the most customisable, supporting virtually any programming language or platform and providing a plugin ecosystem that allows developers to adapt Jenkins to almost any need with the help of tools such as Git, Docker and Kubernetes for a seamless CI/CD pipeline. Travis CI is another popular option, often preferred in the open-source community. It offers a simpler, cloud-based solution that integrates natively with GitHub to simplify CI configuration and automate test execution to the point that it’s often used in small projects or teams that value simplicity over detailed automation.
CircleCI, for example, offers a flexible and highly scalable CI solution. It provides parallel test execution so that tests don’t have to wait for previous ones to complete and customised workflows to help teams design CI processes that maximise speed and efficiency. The tool also integrates with popular version control systems and cloud platforms, which makes it easy to define, configure and manage CI processes even for complex projects. Using these CI tools, developers can automate many repetitive tasks, reduce the amount of time dedicated to manual testing or deployment, and keep the code in a deployable state at all times.
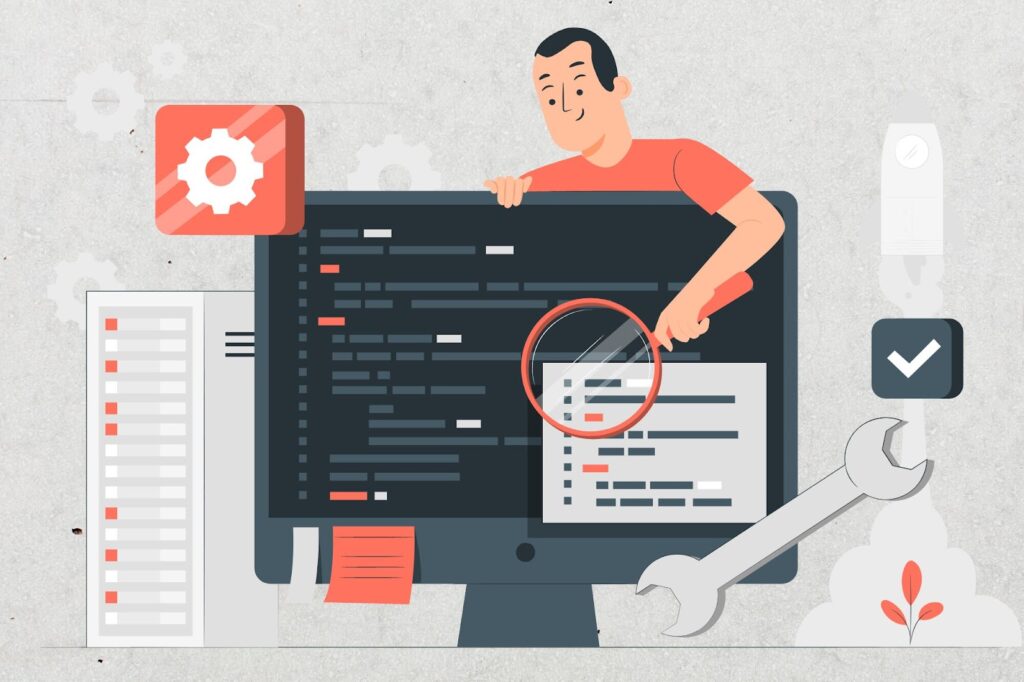
Code Optimisation Techniques
Importance of Code Optimization:
One of the most important aspects of writing software is making sure that it is optimised – written in a way that makes it run fast, use as few resources as possible and be readable, making it easier to maintain and extend as time goes on. In today’s world, where software efficiencies can make or break user experiences, this task is good software practice and a crucial way to gain a competitive advantage. Performance optimisation involves making sure that your application doesn’t choke when it’s under heavy loads. This is especially important for high-traffic websites or real-time systems. Readability involves writing the application in a way that makes it easy for other developers (and your future self) to read and, thus, work with the codebase, reducing the chances of an error being introduced when maintenance or feature development is taking place. Scalability involves ensuring that your software can grow as fast as its user base, scaling with increasing amounts of data and traffic without requiring a major rewrite.
By avoiding code optimisation, developers can create software that not only works slowly and leaks memory, often due to platform limitations, but also is harder to maintain or upgrade. If these issues are allowed to build up over time, it can quickly reach the point where the cost of fixing issues outweighs the benefit of writing new code: this is known as technical debt. By treating optimisation highly, developers can create software that is not only more efficient and reliable but also stands a better chance of survival over time.
Common Optimisation Techniques:
There are many established techniques for improving code performance, each focusing on optimisation and efficiency. One of the earliest and essential techniques is to minimise loops and avoid the use of nested loops, which can dramatically slow execution time. For instance, rather than iterating through a large amount of data multiple times, developers often can rewrite code to accomplish the same thing with a smaller number of iterations, thus reducing the computational overhead.
The second is to reduce memory usage. Developers can do this by choosing the right data structures, eliminating unnecessary data duplication, and freeing memory as soon as it is no longer required. If you go from a lookup table implemented with a list to one using a hash map, that changes from O(n) to O(1), making the thing much faster. Developers can also optimise algorithms to be more efficient in terms of space. Here, optimisation might involve choosing more efficient algorithms (such as a quicksort versus a bubble sort) or optimising existing algorithms by removing redundant operations or simplifying complex calculations.
Other typical optimisations are lazy loading (loading resources on demand) and inlining of code (embedding function code at the call site, which can mitigate function call overheads). There are countless other optimisations that, when applied judiciously, can yield significant positive results, leading to more responsive and less resource-hungry software.
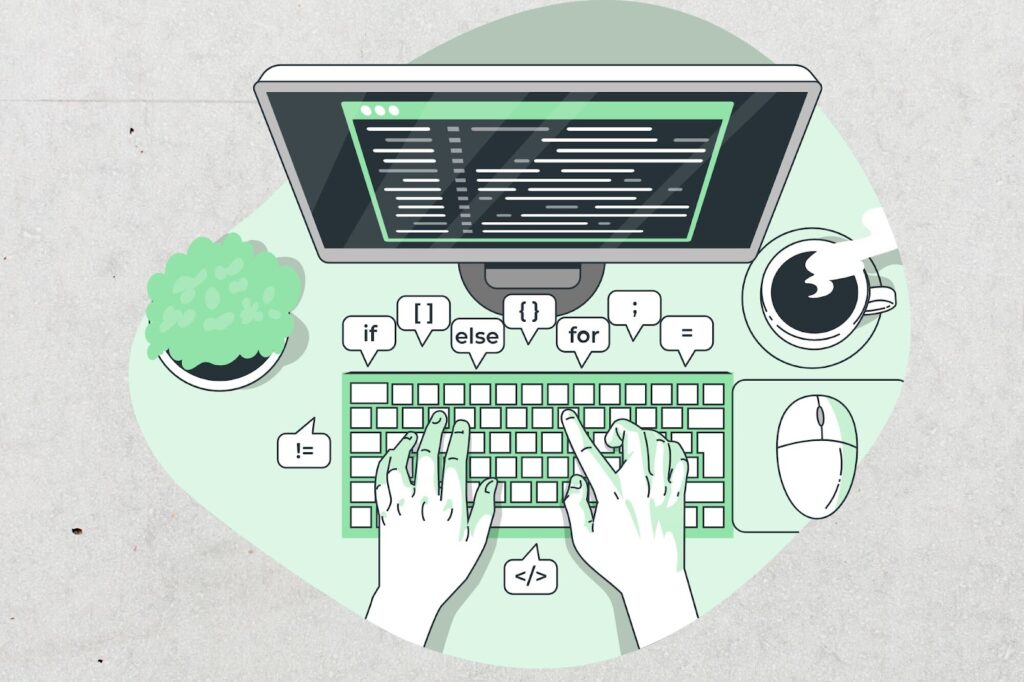
Collaboration and Communication Tools
The Role of Collaboration in Efficient Coding:
Collaboration and communication are key to having a productive codebase, especially in team-based software development environments. It’s common in many projects for many developers to work on different parts of the codebase at once. Therefore, everyone must be on the same page regarding goals, priorities and standards. Good communication helps avoid miscommunication, reduces the risk of conflicting changes, and makes sure everyone knows where the project is and where it’s headed. Without collaboration, even the most capable developers can find themselves working on the same thing, or missing a deadline, or struggling with integration issues.
Sharing code is only one part of collaboration – sharing ideas, knowledge, and feedback are others. As a team, we can leverage the collective experience of everyone involved, leading to better problem-solving, more creative solutions and ultimately, higher-quality code. For example, regular team meetings, code reviews, and even pair programming sessions are all ways to increase collaboration, ensuring that everyone is working towards the same goals and to the same standard.
Top Collaboration Tools:
There are a number of tools that facilitate collaboration and communication: Slack is one of the most popular chat-based communication platforms that enables teams to create channels of conversations related to specific projects or topics. Notifications from other tools, such as GitHub and Jenkins, can be pulled into Slack for real-time updates of events such as code changes, build statuses, etc, so that nobody is left out of the loop.
A second crucial tool is Jira, a project management system that is especially useful in Agile development environments. Jira helps teams track tasks, visualise workflows and monitor progress through customisable dashboards and reports. Its ability to integrate with popular software development tools makes it easy to map code changes to linked tasks or issues, enabling a traceability record from requirements to implementation. Trello is another project-management tool that shows its cards upfront. This tool organises tasks into a card-based system so that teams can set up boards, add members, put deadlines, and track tasks in a clear interface that promotes transparency and accountability.
By applying them, teams are able to communicate more clearly, handle tasks better, and collaborate more efficiently, thereby leading to increased productivity and better project outcomes.
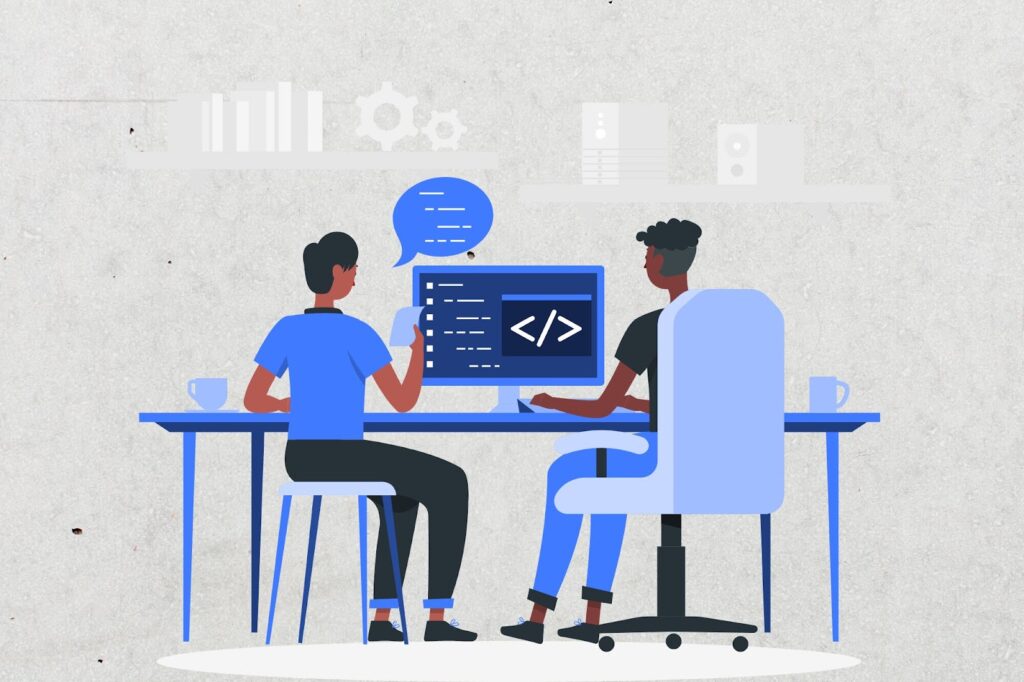
Continuous Learning and Professional Development
The Need for Ongoing Education:
Learning must be continuous, the technology world is ever-changing, and new tools, frameworks, languages, and approaches to development come out almost daily. If a software developer is to keep things efficient and maintain a competitive edge, they must keep up with the changes, expanding their knowledge and skill base. In this way, a developer could use the latest tools and techniques, which may improve the efficiency of their coding, as well as the quality of the software they produce.
Beyond that, a continuous learning approach ensures you are always learning about new challenges and opportunities to apply knowledge to your project. If your project grows in scope and complexity, you can end up with problems you have yet to see before that require creativity to solve. Learning about the latest trends and developments will allow you to approach these challenges using the most up-to-date and effective methods. It will help you meet your goals as quickly and effectively as possible.
Resources for Learning:
Luckily, there are plenty of resources to keep developers learning as they go along. There are online courses on every conceivable aspect of software development, from programming languages and web frameworks to software architecture and DevOps practices, many of which are offered by major providers such as Coursera or Udemy. Many of these courses are taught by true industry experts and, as such, allow developers to further develop their skills by learning from the best in the field and then applying their newfound knowledge to actual work.
Coding boot camps are typically more immersive, with the aim of putting trainees on the job as soon as possible after learning. They often focus on a particular technology stack or methodology and are designed to get developers up to speed on a new area quickly (Le Wagon is a good example, as is General Assembly). If you’re looking to pivot or to advance your career quickly, a boot camp might be the solution.
Developer communities – forums such as Stack Overflow and code repositories such as GitHub – can also be an ongoing source of education. You can ask questions, find answers, and learn from peers and other developers who are eager to collaborate on open-source projects. Participating in these communities can be a great way to stay in the loop, get educated, and give back to the development ecosystem.
Conclusion
The tools and techniques that I mention – from IDEs and version control systems to debugging practices, code reviews and continuous learning – are all ones that you should consider adding to your workflow. Doing so will help you improve your productivity, the quality of your code, and the success of your projects. You can be a better software developer by working more quickly and efficiently, as well as by creating more maintainable and scalable software. Coding efficiency is a lifelong endeavour, and by constantly experimenting with new tools and techniques, you’ll stay ahead of the curve in a field that will never stop changing.